How to use in scenes
All values that are shown or can be modified via the QuickApp are also available externally, for example from a scene. The values can be retrieved with the help of the json object published and periodically updated in the QuickApp’s values variable, while they can be written by calling the set_value() function of the QuickApp with the appropriate parameters. The QuickApp also publishes its own ID in a global variable. You can set the name of this global variable in the QuickApp variable called app_name, e.g.:
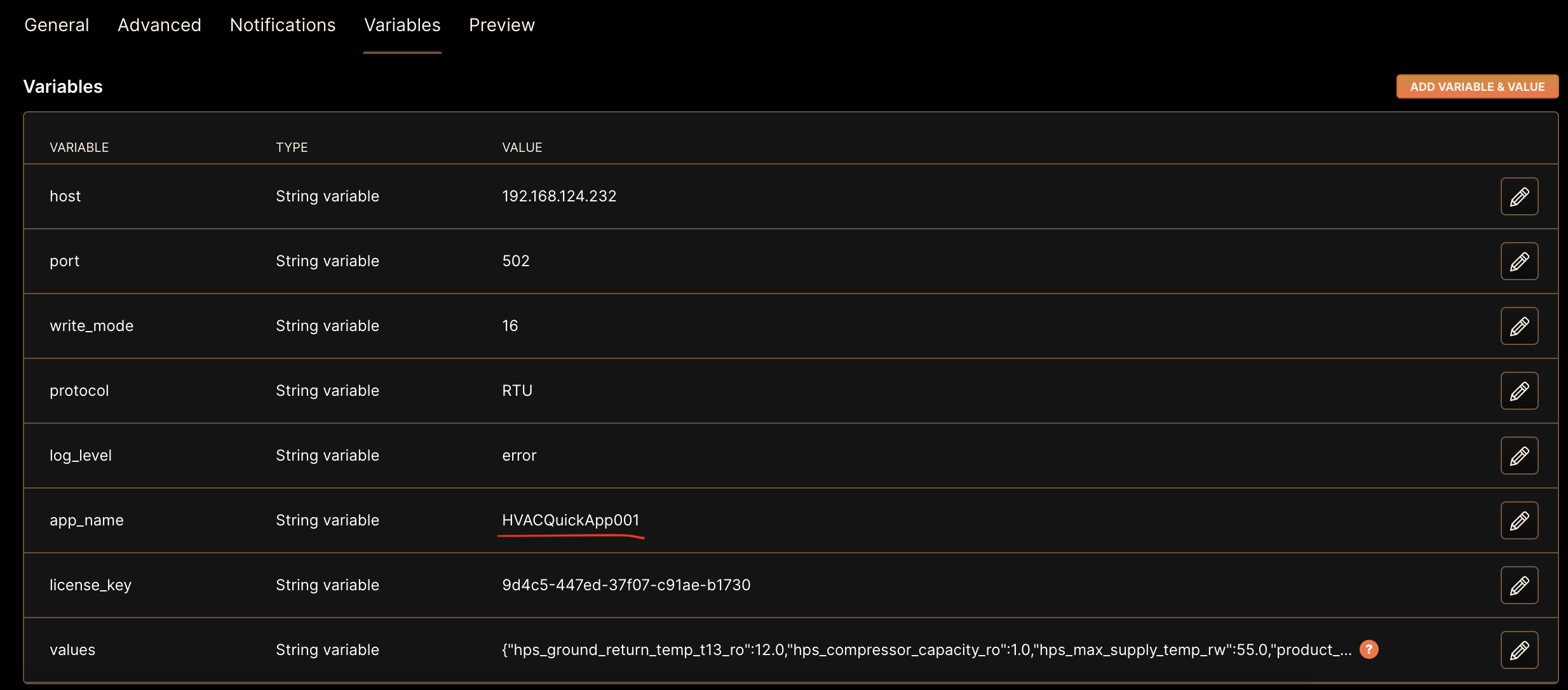
For the above example the global variable called HVACQuickApp001 will contain the ID of the QuickApp that serves the values of your device.
Example
The example scene that implements and demonstrates the above functions can be downloaded here. Feel free to use it, I grant all rights to convert the code and use it for free or resell it. Some explanation of this lua code follows
Dumping objects
This is a helper function that dumps any lua variable in a human readable form
-- Helper function to dump any type of variable
function dump(o)
if type(o) == 'table' then
local s = ''
local sep = ''
for k,v in pairs(o) do
if type(k) ~= 'number' then k = '"'..k..'"' end
s = s .. sep .. k ..': ' .. dump(v)
sep = ", "
end
return '{ ' .. s .. ' }'
elseif type(o) ~= 'number' then
return '"'..tostring(o)..'"'
else
return tostring(o)
end
end
Get/Set QuickApp variables
You can get/set the values of the quickapp by calling the access_var(name, value) function.
local function access_var(name, value)
local QAID = tonumber(hub.getGlobalVariable(app_name))
if not QAID then
print("Error: Rolcsi's QuickApp not found.")
return
end
if (QAVars == nil) or (name == nil) then
local dev = api.get("/devices/"..QAID)
for _,v in ipairs(dev.properties.quickAppVariables) do
if v.name == "values" then QAVars = json.decode(v.value) end
end
end
if name ~= nil then
local old_value = QAVars[name]
if (value ~= nil) and (value ~= old_value) then
hub.call(QAID, "set_value", name, value)
QAVars[name] = value
end
return old_value
end
end
This function can be used in three different ways:
- First of all, if called without any parameters, it will load all the variables of the QuickApp to an associative array called
QAVars
. After this, you cen read e.g. the target temperature value from theQAVars["target_temp"]
variable. - You can call it by specifying only the name parameter for to get the value of a specific variable (this will use the preloaded
QAVars
and updates it only if it has not been loaded yet). - If you want to modify the value of a variable (this will only work for writable variables), you have to specify the name of the variable in the name parameter and its new value in the value parameter, e.g.
old_value = access_var("target_temp", 23.3)
. Then the function will return the previous value of the variable (old_value
).
The reference list of available variables can be found here.